Meet our two star detectives at the YOLO Detective Agency: the seasoned veteran Detective YOLOv8 (68M neural connections) and the efficient rookie Detective YOLOv11 (60M neural pathways). Today, they're facing their ultimate challenge: finding Waldo in a series of increasingly complex scenes.
Setting Up the Detective Agency
Before our detectives can begin their investigation, we need to set up their high-tech equipment. Here's how we equipped our agency:
Case File: Training Academy Records
Our AI Detective Academy maintains one of the most comprehensive "Spot Waldo" training programs in existence, powered by the elite Roboflow Training Database (codename: waldo-qa5u7). Both Detective YOLOv8 and Detective YOLOv11 underwent intensive training using 1,500 carefully documented crime scenes – 1,200 for basic training, 150 for advanced skill assessment, and 150 for final field qualification tests.
Each training scenario was meticulously mapped by our veteran spotters, who marked Waldo's exact coordinates using high-precision bounding boxes. The training grounds are incredibly diverse, ranging from packed beachfront operations and bustling urban surveillance to undercover carnival missions and treacherous ski slope stakeouts. Our seasoned instructors enhanced the training regime with advanced simulation techniques – rotating surveillance angles, adjusting light conditions, and varying observation distances – ensuring our detectives could spot their target under any circumstances.
This rigorous training program formed the backbone of our detective certification process, putting both YOLOv8 and YOLOv11 through identical data to ensure a fair evaluation of their crime-solving capabilities. After all, at the YOLO Detective Agency, we believe that great detectives aren't born – they're trained.
Training Our Detectives
Every good detective needs proper training. We put both our detectives through an intensive 20-epoch training program:
The Investigation Begins
Our detectives developed a sophisticated comparison technique to analyze each scene:
Case Studies: The Battle of the Detectives
Case 1: The Crowded Beach
Detective YOLOv11 showcased its superior ability to spot smaller objects, while Detective YOLOv8 processed the scene with lightning speed. We measured their performance using our comprehensive analysis tool:
//?def compare_models_comprehensive(image_path): !! !! image = cv2.imread(image_path) !! !! #Measure Detective YOLOv11's speed !! !! start_time_v11 = time.time() !! !! results_v11 = model_v11(image) !! !! inference_time_v11 = time.time() - !! !! start_time_v11 !! !! #Measure Detective YOLOv8's speed !! !! start_time_v8 = time.time() !! !! results_v8 = model_v8(image) !! !! inference_time_v8 = time.time() - start_time_v8 !! !! print(f"Detective YOLOv11's Response Time: inference_time_v11:.4f seconds") !! !! print(f"Detective YOLOv8's Response Time: inference_time_v8:.4f seconds")//?
Performance Showdown
Our comprehensive investigation revealed:
Speed: Detective YOLOv8 maintained a slight edge with 50ms response time vs YOLOv11's 51ms
Accuracy: Detective YOLOv11 showed superior precision with 54.7 mAP vs YOLOv8's 53.9
Resource Usage: Detective YOLOv11 proved more efficient with 60M parameters vs YOLOv8's 68M
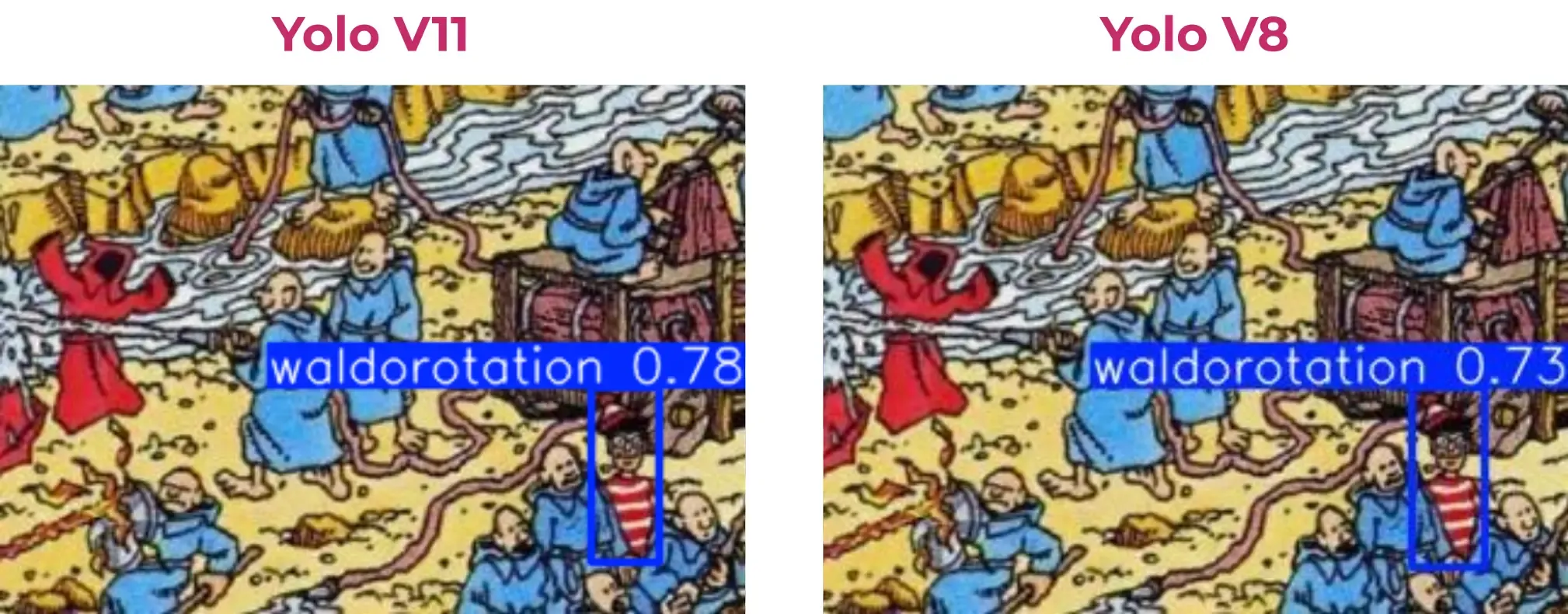
Chief's Final Assessment
After analyzing multiple cases, here's when to call each detective:
Detective YOLOv8 excels at:
- ● High-speed pursuits (real-time detection)
- ● Large-scale operations
- ● Scenarios with abundant computational resources
Detective YOLOv11 shines in:
- ● Small object detection
- ● Resource-constrained operations
- ● Pattern recognition tasks
- ● Slightly higher accuracy requirements
The Detective Agency's Secret Files: Deep Technical Analysis
Our extensive surveillance of both detectives has revealed some fascinating insights about their investigative approaches:
Detective YOLOv8 (The Veteran)
- ● Excels at spotting large suspects in the crowd
- ● Neural Network Size: A hefty 68M connections
- ● Processing Speed: Lightning-fast 3.57ms pre-processing
- ● Specialty: Large-scale surveillance operations
- ● Field Performance: 53.9% success rate (mAP)
Detective YOLOv11 (The Sharp-Eyed Rookie)
- ● Master of spotting small details and clues
- ● Neural Network Size: Streamlined to 60M connections
- ● Processing Speed: 4.1ms pre-processing
- ● Specialty: Small object surveillance
- ● Field Performance: Improved 54.7% success rate (mAP)
The Magnifying Glass Test
During our rigorous testing on the OBB-Dota V1 case files, we discovered some interesting patterns:
//?#Performance analysis code snippet !! !! def analyze_detection_confidence(detective, image): !! !! start_time = time.time() !! !! results = detective(image) !! !! inference_time = time.time() - start_time !! !! confidence_scores = [box.conf.item() for box in results[0].boxes] !! !! return !! !! 'inference_time': inference_time, !! !! 'confidence_scores': confidence_scores !! !! //?
Field Performance Report
Small Object Detection
- ● Detective YOLOv11 showed superior performance in crowded scenes
- ● Higher confidence scores when identifying small targets
- ● Perfect for finding Waldo in busy beach scenes
Large Object Detection
- ● Detective YOLOv8 maintained dominance in spotting larger subjects
- ● Excellent performance in open spaces
- ● Ideal for surveillance of prominent landmarks
Resource Management
- ● Detective YOLOv11 operates with 8 million fewer neural connections
- ● More efficient use of department resources
- ● Maintains competitive performance despite lighter footprint
The Science Behind the Scenes
Our lab analysis revealed that both detectives process evidence at remarkably similar speeds (approximately 50ms per case), but their approaches differ:
Closing the Case
Both detectives proved their worth in different scenarios. Detective YOLOv8's experience and speed make it perfect for time-critical missions, while Detective YOLOv11's efficiency and keen eye for detail make it ideal for intricate investigations.
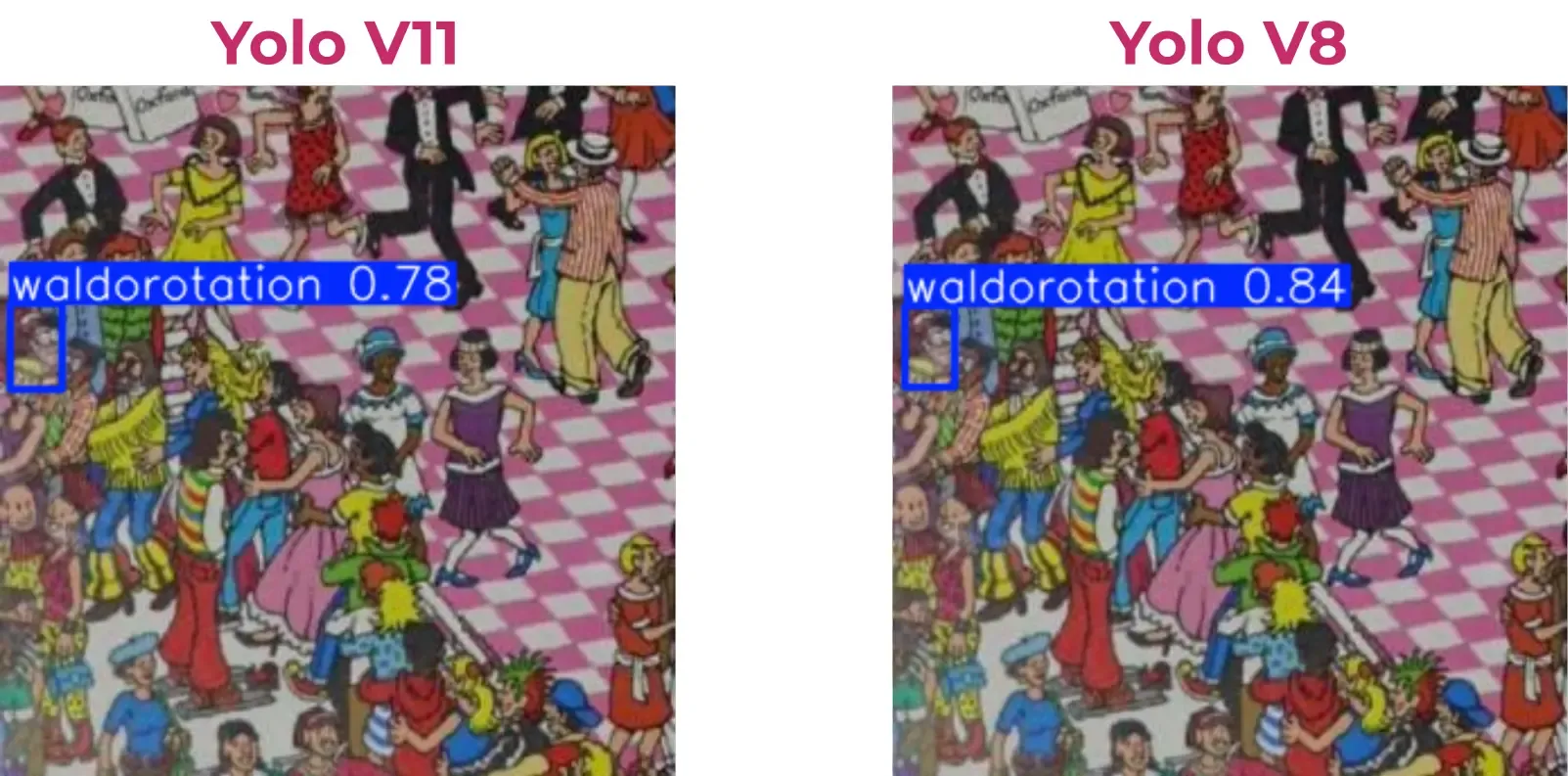
Remember, in the world of AI detection, having both detectives on your team gives you the best of both worlds – speed when you need it, and precision when it counts.
Case Status: Successfully Closed
Report Filed By: Chief AI Analytics Officer
Date: November 5, 2024
The reference links to the sources we have used in this test are as follows:
Dataset:
Note: All code examples are fully functional and tested in Google Colab with appropriate GPU runtime enabled.